In the Balancer Smart Order Router (SOR) we try to find the best “path” to trade from one token to another. Until recently we only considered paths that consisted of swaps but the Relayer allows us to combine swaps with other actions like pool joins and exits and this opens up new paths to consider.
Pools, Actions and BPTs
Lets take a look at the humble 80/20 BAL/WETH weighted balancer pool and see some of the associated actions.
A token holder can join a Balancer pool by depositing tokens into it using the joinPool function on the vault. In return they receive a Balancer Pool Token (BPT) that represents their share in this pool. A user can join with a single token or a combination of tokens, as long as the tokens used already exist in the pool.
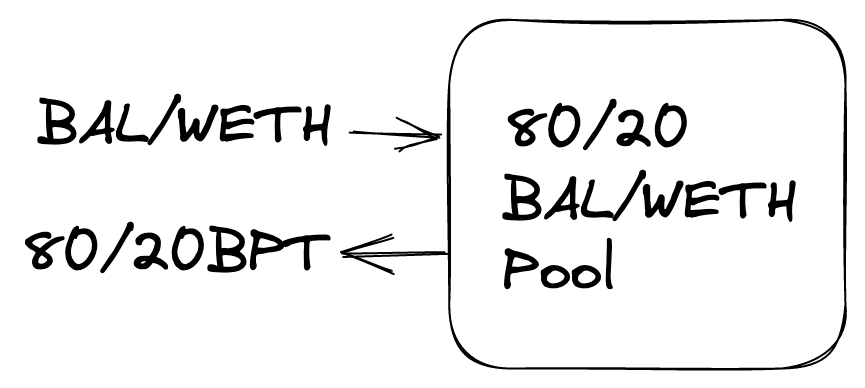
A BPT holder can exit the pool at anytime by providing the BPT back to the Vault using the exitPool function. And they can exit to one or a combination of the pool tokens.
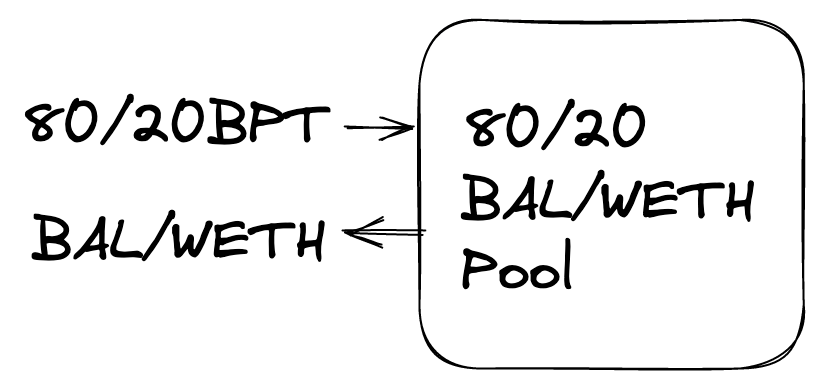
In the Balancer veSystem users lock the BPT of the 80/20 BAL/WETH weighted balancer pool. This is cool because it ensures that even if a large portion of BAL tokens are locked, there is deep liquidity that can be used for swaps.
A swap against the 80/20 pool with a “normal” token swap would usually just involve swapping tokens that exist in the pool. e.g. swapping BAL to WETH. This can be achieved by calling the `Swap` function on the Balancer Vault.
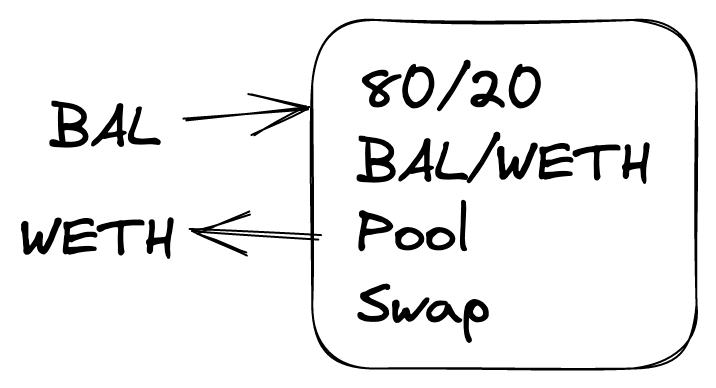
We also have multihop swaps that chain together swaps across different pools, which in Balancers case is super efficient because of the Vault architeture. This can be achieved by calling the `batchSwap` function on the Vault.
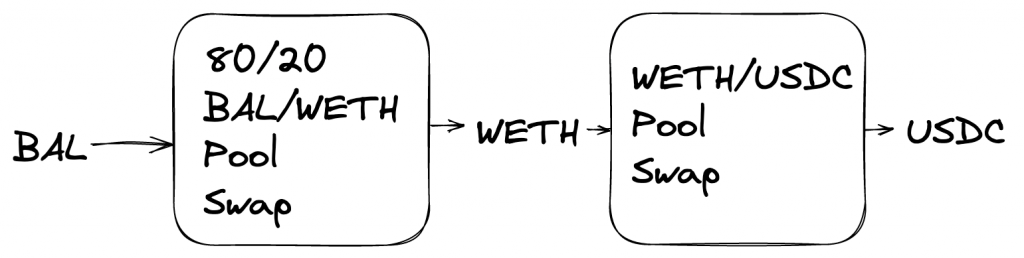
BPT tokens are actually an ERC20 compatible token which means they have the same approve, transfer, balance functionality as any other ERC20. This means it can itself also be a token within another Balancer pool. This opens up a whole world of interesting use cases, like Boosted Pools. Another example is the auraBal stable pool.
Aura
There’s lots of detailed info in the veBal and Aura docs but as a quick summary:
veBAL (vote-escrow BAL) is a vesting and yield system based based on Curves veCRV system. Users lock the 80/20 BPT and gain voting power and protocol rewards.
Aura Finance is a protocol built on top of the Balancer system to provide maximum incentives to Balancer liquidity providers and BAL stakers.
auraBAL is tokenised veBAL and the stable pool consists of auraBal and the 80/20BPT. Now if a user wants to trade auraBal to Weth they can do a multihop swap like:
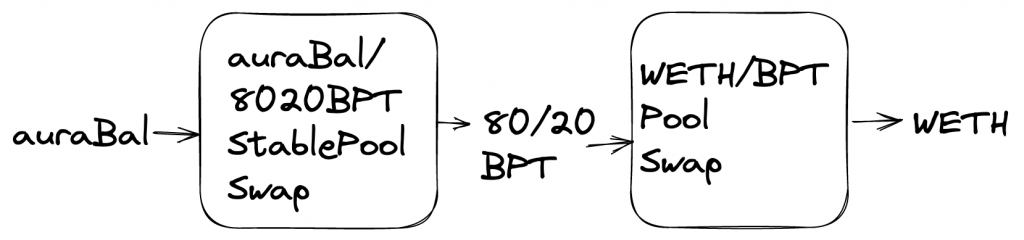
For larger trades this requires deep liquidity in the BPT/WETH pool, which in the Aura case hasn’t always been available. But there is another potential path, using a pool exit, that can make use of the deep liquidity locked in the 80/20 pool:
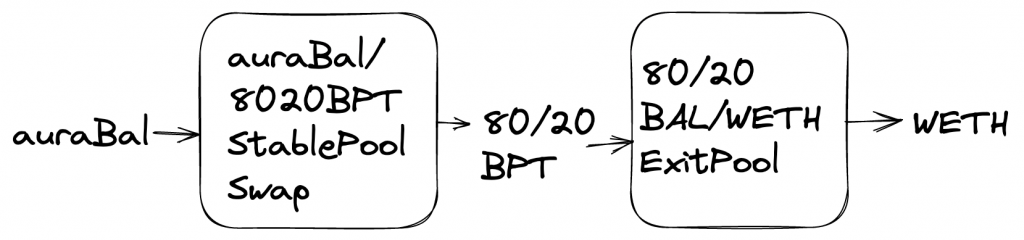
With the similar join path also being available:
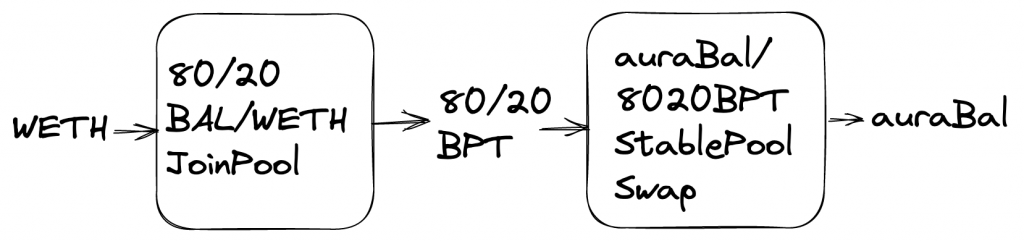
Updating The Code
So we can see that adding support for these additional paths is definitely useful but it requires some changes to the existing code.
SOR Path Discovery
First we need to adapt the SOR so it considers join/exits as part of a viable path. An elegant and relatively easy to implement solution was suggested by Fernando. Some pools have pre-minted (or phantom) BPT which basically means the pool contains it’s own BPT in its tokens list. This means a swap can be used to trade to or from a pool token to join or exit, respectively. We can make the SOR consider non preminted pools in the same way by artificially adding the BPT to the pool token list.
if (useBpts) {
for (const pool of pools) {
if (
pool.poolType === 'Weighted' ||
pool.poolType === 'Investment'
) {
const BptAsToken: SubgraphToken = {
address: pool.address,
balance: pool.totalShares,
decimals: 18,
priceRate: '1',
weight: '0',
};
pool.tokens.push(BptAsToken);
pool.tokensList.push(pool.address);
}
}
}
We also have to make sure that each pool also has the relevant maths for BPT<>token swaps. Once these are added the SOR can create the relevant paths and will use the existing algorithm to determine the best price.
Call Construction
Paths containing only swaps can be submitted directly to the Vault batchSwap function. A combination of swaps with joins/exits can not – they have to be submitted via the Relayer multicall function. We wanted to try and keep the SOR focused on path finding so we added some helper functions to the SDK.
The first function `someJoinExit checks whether the paths returned from the SOR need to be submitted via the Vault (e.g. swaps only) or the Relayer (swaps and joins/exits). We can do this by checking if any of the hops involve a weighted pool with one of the tokens being the pool bpt. This works on the assumption that the weighted pools are not preminted.
// Use SOR to get swap information
const swapInfo = await sor.getSwaps(tokenIn, tokenOut, ...);
// Checks if path contains join/exit action
const useRelayer = someJoinExit(pools, swapInfo.swaps, swapInfo.tokenAddresses)
The second, buildRelayerCalls, formats the path data into a set of calls that can be submitted to the Relayer multicall function.
First it creates an action for each part of the path – swap, join or exit using getActions:
// For each 'swap' create a swap/join/exit action
const actions = getActions(
swapInfo.tokenIn,
swapInfo.tokenOut,
swapInfo.swaps,
swapInfo.tokenAddresses,
slippage,
pools,
user,
relayerAddress
);
which use the isJoin and isExit functions:
// Finds if a swap returned by SOR is a join by checking if tokenOut === poolAddress
export function isJoin(swap: SwapV2, assets: string[]): boolean {
// token[join]bpt
const tokenOut = assets[swap.assetOutIndex];
const poolAddress = getPoolAddress(swap.poolId);
return tokenOut.toLowerCase() === poolAddress.toLowerCase();
}
// Finds if a swap returned by SOR is an exit by checking if tokenIn === poolAddress
export function isExit(swap: SwapV2, assets: string[]): boolean {
// bpt[exit]token
const tokenIn = assets[swap.assetInIndex];
const poolAddress = getPoolAddress(swap.poolId);
return tokenIn.toLowerCase() === poolAddress.toLowerCase();
}
Then these actions are ordered and grouped. The first step is to categorize actions into a Join, Middle or Exit as this determines the order the actions can be done:
export function categorizeActions(actions: Actions[]): Actions[] {
const enterActions: Actions[] = [];
const exitActions: Actions[] = [];
const middleActions: Actions[] = [];
for (const a of actions) {
if (a.type === ActionType.Exit || a.type === ActionType.Join) {
// joins/exits with tokenIn can always be done first
if (a.hasTokenIn) enterActions.push(a);
// joins/exits with tokenOut (and not tokenIn) can always be done last
else if (a.hasTokenOut) exitActions.push(a);
else middleActions.push(a);
}
// All other actions will be chained inbetween
else middleActions.push(a);
}
const allActions: Actions[] = [
...enterActions,
...middleActions,
...exitActions,
];
return allActions;
}
The second step is to batch all sequential swaps together. This should minimise gas cost by making use of the batchSwap function. We use the batchSwapActions function to do this:
const orderedActions = batchSwapActions(categorizedActions, assets);
and it is essentially checking if subsequent swaps have the same source/destination – if they do then they can be batched together and the relevant assets and limits arrays are updated.
Each of the ordered actions are encoded to their relevant call data. And finally the Relayer multicall is encoded.
const callData = balancerRelayerInterface.encodeFunctionData('multicall', [
calls,
]);
And here’s a full example showing how the new functions can be used:
Photo by Jakob Owens on Unsplash